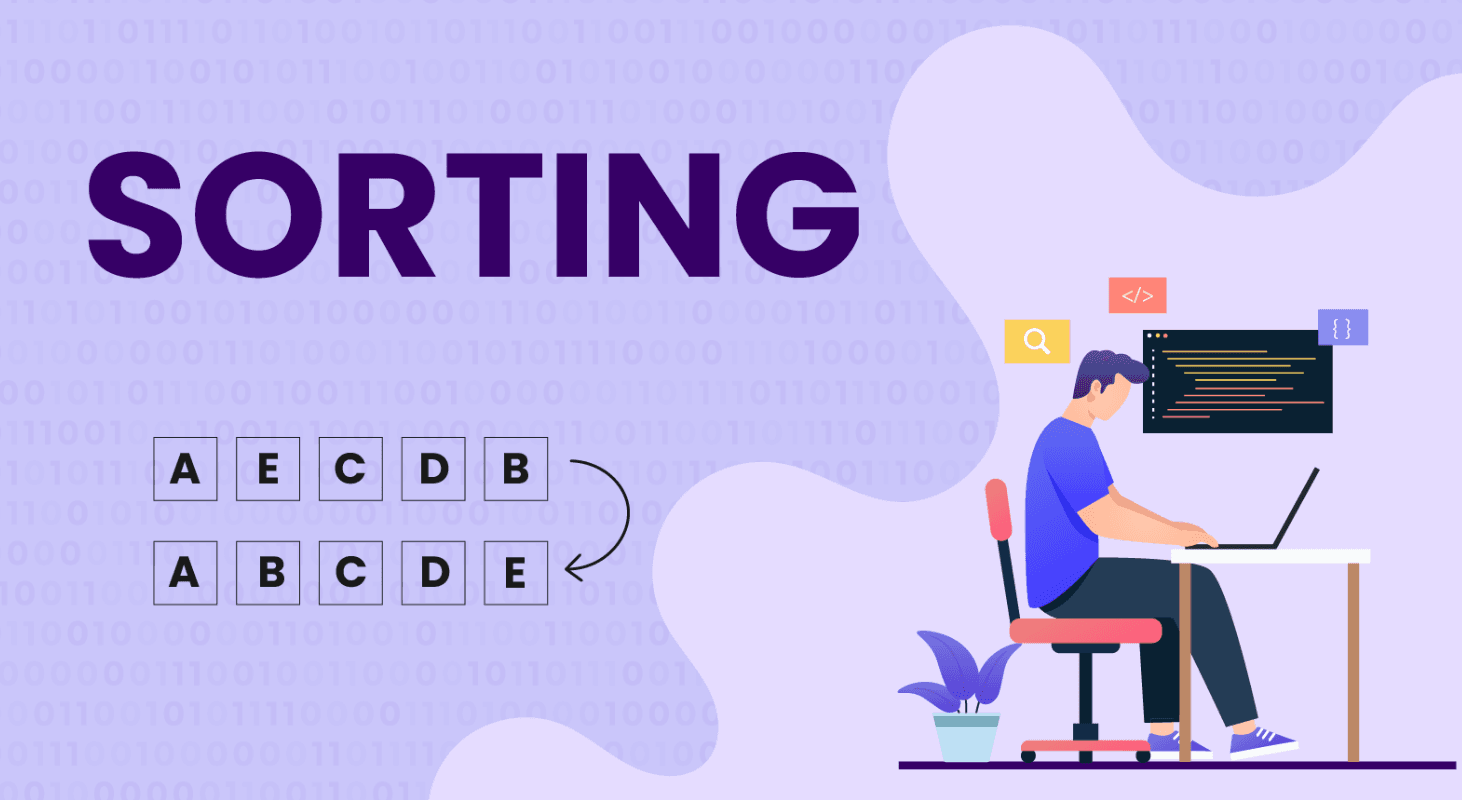
Sorting Algorithms In JS
13 February 2023

Bibhabendu
Sorting Algorithms is very important and interesting concept for every developers.
DBMS
Sorting Algorithm
A sorting algorithm is a process of arranging the elements of an array or a list in a particular order, such as increasing or decreasing order based on a specific comparison function. Sorting is an important operation in computer science and is used in many applications such as searching, data analysis, and data compression.
- Bubble Sort: This is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order.
function bubbleSort(arr) {
// Implement bubblesort
for (let i = 0; i < arr.length; i++) {
for (let j = 0; j < (arr.length - i - 1); j++) {
if (arr[j] > arr[j+1]) {
const lesser = arr[j+1];
arr[j+1] = arr[j];
arr[j] = lesser;
}
}
}
// return the sorted array
return arr;
}